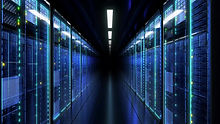

Âncoras
Coisas de Python
Historia
Python was conceived in the late 1980s [43]by Guido van Rossum at Centrum Wiskunde & Informatica (CWI) in the Netherlands as a successor to the ABC programming language, which was inspired by SETL, [44]exception-handling and interface with the Amoeba operating system.[13] Its implementation began in December 1989.[45] Van Rossum assumed sole responsibility for the project, as the lead developer, until July 12, 2018, when he announced his "permanent leave" from his responsibilities as the "benevolent dictator of Python "for life", a title that the Python community awarded him to reflect his long-term commitment as the project's lead decision maker.In January 2019, active Python core developers elected a five-member Board of Directors to lead the project.
Python is a multiparadigm programming language. Object-oriented programming and structured programming are fully supported, and many of its features support functional programming and aspect-oriented programming (including metaprogramming and metaobjects).[67] Many other paradigms are supported through extensions, including contract design [68][69] and logic programming.
Python uses dynamic typing and a combination of reference counting and a cycle-detection garbage collector for memory management. It uses dynamic name resolution (late binder), which binds method and variable names during program execution.
Its design offers some support for functional programming in the Lisp tradition. It has filter,, , - A , standing on what about what about, about what about what about, , about what about what about, about what about what about mapAnd the reduce functions; list comprehensions, dictionaries, groups, and generative expressions. The standard library has two modules ( itertools and functools) that implement functional tools borrowed from Haskell and standard ML
Zen of Python:
The principles are listed as follows:
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.[a]
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than right now.[b]
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea – let's do more of those!
Tkinter(TK)
This was my first original work in graphical mode GUI with python.
Code:
'''
def troca_fonte(event):
"Muda a fonte dada baseada na dropbox ."
if font_option.get() == 'none':
my_font = (font_family.get(), font_size.get())
else:
my_font = (font_family.get(), font_size.get(), font_option.get())
#Muda o estilo de texto
txt_edit.config(font=my_font)
def novo_file():
"Criar um novo ficheiro limpando o screen."
question = messagebox.askyesno("Novo documento!", "Tem a certeza que quer criar um novo ficheiro?")
if question == 1:
#ScrolledText widgets começam no index 1.0 e não 0.
txt_edit.delete("1.0", END)
win.title('Novo Ficheiro - Meus Eventos')
status_bar.config(text='Novo Ficheiro')
global open_status_name#acrescentei esta linha para salvar
open_status_name = False
def fechar_file():# esta função podia ser evitada com o command=win.quit
"Fecha o ficheiro e sai do programa."
#Use a messagebox para perguntar se quer fechar
question = messagebox.askyesno("Feche o ficheiro ", "Tem a certeza que quer fechar o ficheiro??")
if question == 1:
win.destroy()
def salvar_file():
global open_status_name
if open_status_name:
text_file = open(open_status_name, 'w')
text_file.write(txt_edit.get(1.0, END))
text_file.close()
status_bar.config(text=f'Saved: {open_status_name}')
else:
salvar_como()
global open_status_name #acrescentei esta linha para a função salvar
open_status_name = False # esta
def salvar_como():
text_file = filedialog.asksaveasfilename(defaultextension='.*', initialdir="./", title="Salvar o evento", filetypes=(("Documentos de texto", "*.txt"),('Todos os ficheiros','*.*')))
if text_file:
nome = text_file
status_bar.config(text=f'Saved: {nome}')
nome = nome.replace('./', "")
win.title(f'{nome} - Os meus eventos')
text_file = open(text_file, 'w')
text_file.write(txt_edit.get(1.0, END))
text_file.close()
def abrir_file():
txt_edit.delete("1.0", END)
open_name = filedialog.askopenfilename(initialdir="./", title='Abrir apontamento', filetypes=(("Text Files", "*.txt"), ("All Files", "*.*")))
if open_name: #esta
global open_status_name#acrescentei esta linha para salvar
open_status_name = open_name#esta
nome = open_name
status_bar.config(text=f'{nome}')
nome = nome.replace('./', '')
win.title(f'{nome} - Os meus eventos')
open_name = open(open_name, 'r')
conteudo = open_name.read()
txt_edit.insert(END, conteudo)
open_name.close()
'''
#------------funções--código-----
from tkinter import *
from tkinter import ttk
from tkinter import StringVar, IntVar, scrolledtext, END, messagebox, filedialog
from tkinter import font
win = Tk()
win.title('Os meus eventos!')
win.iconbitmap('imagens/fg.ico')
#win['bg'] = 'LimeGreen'
#win.geometry('700x400')
#-----------------centraliza a janela
largura = 700
altura = 700
#resolução do nosso sistema
larg_screen = win.winfo_screenwidth()
alt_screen = win.winfo_screenheight()
#posição da janela
posx = larg_screen/2 - largura/2
posy = alt_screen/2 - altura/2
#defenir a geometry
win.geometry('%dx%d+%d+%d'%(largura, altura, posx, posy))
#---------------------
#win.resizable(width=False, height=False)
#win.resizable(0,0)
win.rowconfigure(0, minsize=500, weight=1)
#tem que ter estes dois valores para se expandir no total e ser responsivo
win.columnconfigure(1, minsize=500, weight=1)
#--------------------------------------
#Definição de fonts e cores
text_color = "#fffacd"
win_color = "white"
win.config(bg=win_color)
fonte = ('Helvetica',25, 'normal')
fonte1 = ('Helvetica',17, 'bold')
txt_edit = Text(win, font= fonte, bg=text_color, selectbackground='yellow', selectforeground='black', undo=True,)
txt_edit.focus()
relevo = 'raised'
fram_buttons =Frame(win, width=100, relief=relevo, bd=2)
fram_buttons.configure(bg='Sienna',)
btn_novo = Button(fram_buttons, text="Novo", font= fonte1, command = novo_file)
btn_open = Button(fram_buttons, text="Abrir", font= fonte1, command = abrir_file)
btn_saveas = Button(fram_buttons, text="SalvarC ", font= fonte1, command = salvar_como)
btn_save = Button(fram_buttons, text="Salvar ", font= fonte1, command = salvar_file)
btn_fechar = Button(fram_buttons, text="Fechar", font= fonte1, command = fechar_file) # = a command=win.quit, sem função
btn_novo.configure(bg='SaddleBrown', bd=5) # a largura do botão é dada pela largura da palavra maior
btn_open.configure(bg='SaddleBrown', bd=5)
btn_saveas.configure(bg='SaddleBrown', bd=5)
btn_save.configure(bg='SaddleBrown', bd=5)
btn_fechar.configure(bg='SaddleBrown', bd=5)
btn_novo.grid(row=0, column=0, sticky="ew", padx=5, pady=3)
btn_open.grid(row=1, column=0, sticky="ew", padx=5, pady=3)
btn_saveas.grid(row=2, column=0, sticky="ew", padx=5, pady=3)
btn_save.grid(row=3, column=0, sticky="ew", padx=5, pady=3)
btn_fechar.grid(row=4, column=0, sticky="ew", padx=5, pady=3)
fram_buttons.grid(row=0, column=0, sticky="ns") #1ªcoluna,primeira linha
txt_edit.grid(row=0, column=1, sticky="nsew") #2ªcoluna
families = ['Fontes', 'Arial', 'Arial Black', 'Calibri', 'Cambria', 'Comic Sans MS', 'Courier', 'Helvetica','Gabriola',
'Georgia', 'Marlett', 'Modern', 'MS Gothic', 'Script','SimSun', 'Tahoma', 'Times New Roman',
'Ubuntu Condensed' 'Verdana', 'Wingdings',]
font_family = StringVar()
font_family_drop = OptionMenu(fram_buttons, font_family, *families, command=troca_fonte)
font_family.set('Fontes')
font_family_drop.config(bg='SaddleBrown', bd=5)
font_family_drop.grid(row=5, column=0, sticky="ew", padx=5, pady=3)
sizes = [8, 10, 12, 14, 16, 20, 25, 32, 48, 64, 72, 96]
font_size = IntVar()
font_size_drop = OptionMenu(fram_buttons, font_size, *sizes, command=troca_fonte)
font_size.set(25)
font_size_drop.config(bg='SaddleBrown', bd=5)
font_size_drop.grid(row=6, column=0, sticky="ew", padx=5, pady=3)
options = ['normal', 'bold', 'italic', "underline"]
font_option = StringVar()
font_option_drop = OptionMenu(fram_buttons, font_option, *options, command=troca_fonte)
font_option.set('normal')
font_option_drop.config(bg='SaddleBrown', bd=5)
font_option_drop.grid(row=7, column=0, sticky="ew", padx=5, pady=3)
#Layout do frame texto
my_font = (font_family.get(), font_size.get())
scroll = Scrollbar(win, command=txt_edit.yview)
txt_edit.configure(yscrollcommand=scroll.set)
scroll.grid(row=0, column=2, sticky="ns")
status_bar = Label(win, text = 'Pronto')
status_bar.grid(row=1, columnspan=4,)
win.mainloop()
Objective: Create a software with python
After having classes on widgets in TK, and of course programming in Python up to a level considered medium, without building a complete 'software' as an example this was a difficult project to develop, done individually and also being my first program with a complete GUI, it was a challenge at the end of the course, but as the challenge was overcome, I gladly share this work.
Sites Uteis >>>
